Java反射中getGenericInterfaces和getInterfaces的解读
今天在做解析网络请求后得到的数据的转化的时候用到了:getGenericInterfaces这个方法。
/**
* 获取回调接口中 T 的具体类型
*
* @param clazz
* @return
*/
public static Type getTType(Class clazz) {
//以Type的形式返回本类直接实现的接口.
Type[] types = clazz.getGenericInterfaces();
if (types.length > 0) {
//返回表示此类型实际类型参数的 Type 对象的数组
Type[] interfacesTypes = ((ParameterizedType) types[0]).getActualTypeArguments();
return interfacesTypes[0];
}
return null;
}
其中回调接口为:
new RequestListener <> () { |
类RequestListener为:
public interface RequestListener { |
使用Gson进行json的解析,T fromJson(String json, Type typeOfT);那么怎么才能获取到RequestListener中的的类型呢?
于是我们从接口获取参数化类型处理。
官方文档解释
getGenericInterfaces:
Returns the {@code Type}s representing the interfaces directly implemented by the class or interface represented by this object.释意:返回表示由此对象表示的类或接口直接实现的接口的{@code Type}。
getInterfaces:
Determines the interfaces implemented by the class or interface represented by this object.
释意:返回由此对象表示的类或接口实现的接口。
从解释上面来看出来了,差异在于“接口实现的接口的Type”,接下来用具体示例来解释区别
private class Food{ |
运行结果
{% qnimg 245442107557694aef0f07c25be0740187c.jpg %}
我们可以看到,clazz.getGenericInterfaces()与clazz.getInterfaces()并没有任何差异。因为 并没有:“实现的接口的Type”
接下来看另一段代码,我们对Eat接口改造一下,增加一个参数化类型
private class Food{
String foodName;
}
private interface Eat{
void eat(T things);
}
private interface Run{
void run();
}
private class Dog implements Eat,Run{
@Override
public void run() { }
@Override
public void eat(Food things) { }
}
private void main() {
Class clazz = Dog.class;
Type[] genericInterfaces = clazz.getGenericInterfaces();
Class[] interfaces = clazz.getInterfaces();
}
运行结果:
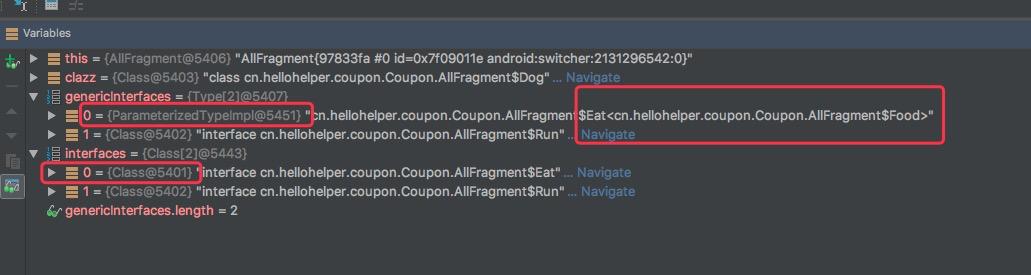